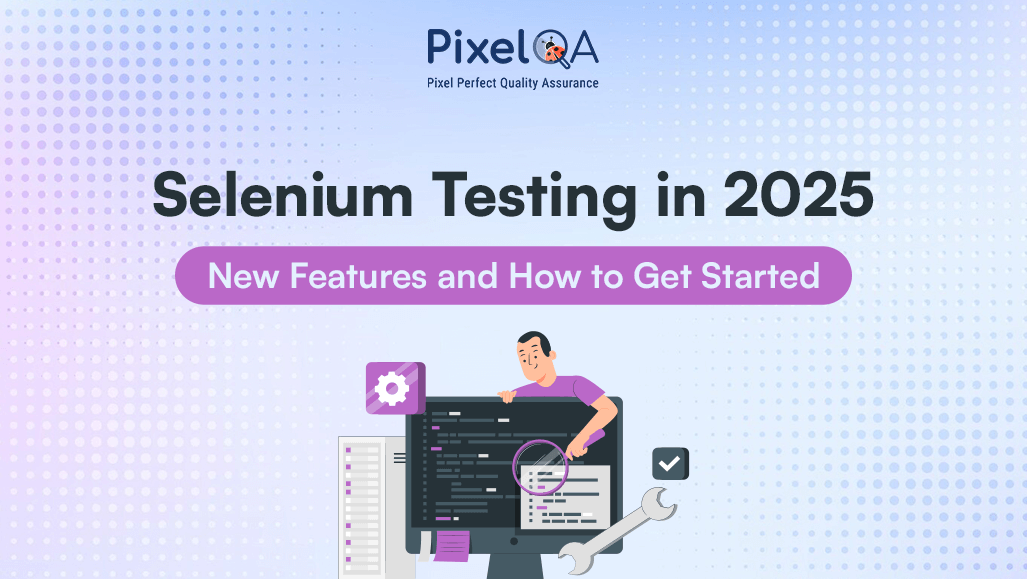
Table of Content
Introduction
Selenium continues to lead automation tools to automated web applications in Test Automation Services, it has enhancements and introduced new features that improve Selenium functionality and user experience. it continues to evolve, adapting to the changing landscape of web technologies, automation needs, and developer practices. While the basic essence behind Selenium remains the same in Automation Testing Services, its role and functionality have expanded, driven by the rise of modern web applications, new browser technologies, and the growing demand for robust, scalable test automation frameworks. Its ability to drive real browsers (Chrome, Firefox, Edge, etc.) across multiple platforms (Windows, macOS, Linux) and it has evolved alongside browser updates, making it compatible with the latest versions of all major browsers. Its compatibility with programming languages such as Java, Python, C#, JavaScript allows it to integrate into diverse development ecosystems. Here, what is new and how to get started with Selenium.
New Features in Selenium
1. Enhanced Browser Support:
- Improved compatibility for latest versions of browsers Firefox, Chrome, and Edge.
- Introduction for support of new browser technologies, such as WebKit for Safari.
2. Selenium Grid 4 Enhancements:
- Better resource management and faster execution of tests across multiple machines.
- Improved support for containerized environments, allowing for seamless integration with tools like Docker and Kubernetes.
3. New API Features:
- Simplified APIs for common tasks, reducing the amount of code required to perform actions.
- Support for asynchronous testing, allowing tests to run in a more efficient, non-blocking manner.
4. Visual Testing Integration:
Built-in support for visual testing frameworks, enabling users to verify that web pages render correctly across different browsers and devices.
5. Advanced Reporting Capabilities:
- Enhanced reporting features that provide detailed insights and analytics on test execution.
- Support for integrating third-party reporting tools.
6. Improved Documentation and Tutorials:
- More comprehensive resources, including interactive tutorials and community-driven content, to help new users get started quickly.
Getting Started with Selenium
1. Set Up Your Environment and Create New Project:
Checklist to ensure the following prerequisites installed:
Java Development Kit (JDK):
- Download and install the latest version of JDK compatible with your operating system from the website Java Downloads | Oracle.
- Add java bin directory path in environment variables, Open environment variables(from control panel) locate path and add the JDK location in the path and save changes.
- In Command prompt Use the command “ java – version” to verify java installation.
Integrated Development Environment (IDE): Choose an IDE such as Eclipse and install it. (for Eclipse download from https://www.eclipse.org/downloads/)
Selenium WebDriver: Download the Selenium WebDriver with Java (if you choose Selenium with Java) from the official Selenium website (https://www.selenium.dev/downloads/) or include it as a dependency in your project using tools like Maven or Gradle.
Launch Eclipse IDE and create a new Java/Maven project. Configure the project settings and ensure you’ve added the Selenium WebDriver library to your project’s classpath.
2. Start with Selenium Basics:
- Start with basic selenium concepts including elements, locators, actions. (https://www.javatpoint.com/selenium-webdriver-commands).
3. Write Test script:
- Start with simple test scripts to navigate to a website and interact with webpage elements and assert expected outcomes.
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class Test{
public static void main(String[] args) {
// Set the path for the ChromeDriver
System.setProperty("webdriver.chrome.driver", "path/chromedriver");
// Create a new instance of the Chrome driver
WebDriver driver = new ChromeDriver();
try {
// Navigate to a website
driver.get("https://www.example.com");
// Get the page title
String pageTitle = driver.getTitle();
// Expected title
String expectedTitle = "PageTitle";
// Verify the page title
if (pageTitle.equals(expectedTitle)) {
System.out.println("Page title is verified successfully: " + pageTitle);
} else {
System.out.println("Page title is not verified as expected. " + expectedTitle );
}
WebElement inputField = driver.findElement(By.name("inputfieldName"));
inputField.sendKeys("Hello, World!");
// Example of clicking a button
WebElement button = driver.findElement(By.id("submitbuttonId"));
button.click();
// Example of selecting an option from a dropdown
WebElement dropdown = driver.findElement(By.id("dropdownId"));
dropdown.click();
// Click to open the dropdown
WebElement option = driver.findElement(By.xpath("//option[text()='option text']"));
option.click();
// Click the desired option
// Example of checking a checkbox
WebElement checkbox = driver.findElement(By.id("checkboxId"));
if (!checkbox.isSelected()) {
checkbox.click();
// Click to select if not already selected
}
// Example of getting text from an element
WebElement messageElement = driver.findElement(By.id("messageelementId"));
String message = messageElement.getText();
System.out.println("Message displayed: " + message);
}
}
} finally {
// Close the browser
driver.quit();
}
}
}
- Gradually add more scenarios like pop-ups, handling alerts and asynchronous operations.
4. Use parallel test execution:
- Set up parallel test execution to save time. Refer this blog for process: (Parallel Execution for Selenium Test Suites in Java)
5. Integrate with CI/CD Pipelines:
- Integrate Selenium tests with continuous integration/continuous deployment (CI/CD) tools like CircleCI , Jenkins, or GitHub Actions.
- Automate test execution process to run tests with every build or deployment.
6. Add Advanced Features:
- Add reporting tools to enhance your testing suite.
Conclusion
Selenium offers vigorous features for web application testing, making it easier than ever to make sure applications work as intended on different browsers and devices. By using the latest updates and resources, enhancing testing processes using Selenium Automation Testing Services and delivering high-quality software.
About Author
Jigna Jadav started her journey as a QA executive in manual testing and moved her career up to QA Team Leader. She aspires to train and mentor her juniors and enhance the automation infrastructure.