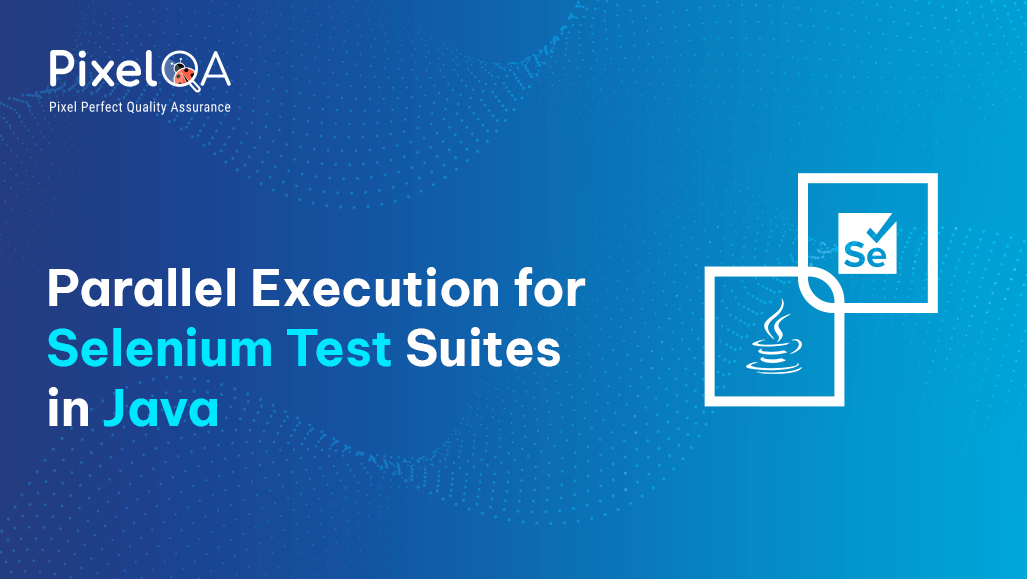
In software development, one thing is paramount: agility and quality go hand in hand. In order to be agile, testing processes must be efficient. Selenium provides the great advantage of testing automation for web-based applications. Selenium automation testing services enable developers and QA teams to improve the efficiency of testing. And as applications become more complex, testing takes much longer. For this, sometimes the situation itself is not peaceful. It just happens that parallel execution comes in the picture, shining like a beacon of efficiency as multiple tests run concurrently in order to cut down the actual execution time.
Table of Contents
- Understanding the Essence of Parallel Execution
- Navigating the Setup Terrain
- A Step-by-Step Guide
- Advantages
- Considerations and Best Practices
- Conclusion
Understanding the Essence of Parallel Execution
Although the standard sequential execution of Selenium tests works fine for smaller test suites, it does not work well for larger, more complex applications. Instead, you want to test in parallel. Parallel Testing brings a different thought process to testing since it allows tests to run simultaneously or in parallel with other processes while making maximum use of available computing resources.
Selenium supports the parallel execution of tests by launching tests in separate thread processes. This means you can run multiple tests at the same time on multiple devices, browsers or scenarios instead of simply executing the tests sequentially. Thus, with parallel execution, test execution time is significantly reduced and test efficiencies improved.
You can distribute test cases over several threads or machines and enable simultaneous test execution by utilizing Selenium's parallel execution feature. To prevent tests from interfering with one another, each test runs independently using different browser instances or devices.
Large test suites or testing across multiple configurations benefit greatly from parallel execution. It improves test coverage, makes the most use of available resources, and delivers test result feedback more quickly.
Navigating the Setup Terrain
Before delving into the implementation of parallel execution, it's essential to lay the groundwork:
- Selenium WebDriver Setup: Install and configure Selenium WebDriver along with the supporting browser drivers suitable for your testing environment.
- Testing Framework Selection: Choose a good testing framework that can be easily used for parallel execution. Of course, it would be best to go for TestNG or JUnit, which are strongest in Java for the support of parallelism.
- IDE or Build Tool Integration: Use any of them, such as IntelliJ IDEA, Eclipse, Maven, or Gradle, to manage dependencies and execute the tests.
Implementation Unveiled: A Step-by-Step Guide
It would be interesting for us to explore the implementation of parallel execution of TestNG test suites, that is one of the most favorite testing frameworks used by Java experts:
- Test Method Grouping: Organize your test methods into logical groups using TestNG's `@Test` annotation and the `groups` attribute. This delineates the boundary for parallel execution.
Example:
@Test(groups = "group1")
public void testMethod1() {
// Test logic
}
@Test(groups = "group2")
public void testMethod2() {
// Test logic
}
- TestNG XML Configuration: Craft a TestNG XML file where you orchestrate the suite, test, and parallel execution settings. Although it is reasonably evident that parallel testing must be used with the test case methods to run them in parallel TestNG offers three more areas where we can go ahead with parallel testing, combining these four areas, parallel testing accepts the following keywords (values) in TestNG:
- Methods: All of the @Test methods in TestNG will have their parallel tests executed by this.
- Tests: This value will be used to execute each test case contained within the element.
- Classes: Every test case that is contained in an XML class will execute parallelly.
- Instances: Using this value, a single instance will be used to run each test case parallelly.
Sample XML configuration:
<!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd">
<suite name="Parallel Test Suite" parallel="tests" thread-count="5">
<test name="Test">
<groups>
<run>
<include name="group1"/>
<include name="group2"/>
</run>
</groups>
<classes>
<class name="com.example.TestClass"/>
</classes>
</test>
</suite>
- Test Configuring to Execute in Parallel in TestNG: Using the Selenium web driver, execute the test suites, classes, and methods in parallel in TestNG. To indicate to TestNG how many threads we would like to create, it also included mentioning the thread-count. However, by defining the test method inside the test code itself, TestNG also gives us the freedom to run a single test method in parallel. We accomplish this by tweaking the @Test annotation a little bit. Take note of the parameters in the @Test annotation and the code that follows.
public class Test
{
@Test(threadPoolSize = 3, invocationCount = 3, timeOut = 2000)
public void test()
{
System.out.println("Thread ID Is : " + Thread.currentThread().getId());
}
}
Three arguments need to be mentioned in the @Test annotation:
- threadPoolSize: The number of threads we want to start and use to execute the test in parallel.
- invocationCount: The desired number of invocations for this particular method.
- timeOut: The longest period of time that a test should run. The test automatically fails if the limit is exceeded.
- Parallel test execution in TestNG with DataProviders: TestNG allows parallel testing execution with DataProviders, hence running multiple test case in a single-fell swoop using different sets of data. This method can simply be used by configuring the parallel attribute in the @DataProvider annotation as well as setting the parallelism in the TestNG XML file, hence providing an efficient resource utilization and a reduction in the execution time as well as performance.
Create a DataProvider with parallel execution:
public class TestData {
@DataProvider(name = "data-provider", parallel = true)
public Object[][] dataProviderMethod() {
return new Object[][] { { "data1" }, { "data2" }, { "data3" } };
}
}
- Use the DataProvider in a Test Method:
public class TestClass {
@Test(dataProvider = "data-provider", dataProviderClass = TestData.class)
public void testMethod(String data) {
System.out.println("Test method executed with: " + data + " by " + Thread.currentThread().getName());
}
}
- Configure parallel execution in TestNG XML:
<!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd">
<suite name="ParallelTests" parallel="methods" thread-count="3">
<test name="Test">
<classes>
<class name="TestClass"/>
</classes>
</test>
</suite>
This setup ensures that the test method runs in parallel for different data sets provided by the Data Provider.
- Test Execution Command: Execute the orchestrated tests using TestNG, specifying the path to the TestNG XML file. For adherents of Maven, integrate the TestNG plugin into your `pom.xml` and initiate test execution via the command line.
mvn test -DsuiteXmlFile=testng.xml
Unveiling the Advantages: A Panorama of Benefits
The adoption of parallel execution confers a plethora of benefits upon testing endeavors:
- Faster Test Execution: Parallel testing cuts down on the total amount of time required to finish tests. This expedites the release process and provides faster feedback.
- Better Resource Use: Parallel execution uses computing resources more efficiently. This ensures that available hardware is used well and increases testing output.
- Seamless Scalability: Parallel execution maintains performance consistency regardless of size or complexity as the test suite grows and changes.
Navigating the Terrain: Considerations and Best Practices
While parallel execution unlocks unparalleled efficiency, it's imperative to navigate certain considerations judiciously:
- Thread Safety Awareness: Maintaining thread safety within the test code is critical to avoid data corruption or unexpected side effects created by concurrent test running.
- Resource Management Awareness: Judicious resource management principles are critical to prevent resource contention situations, especially where tests engage with outside systems or resources.
- Sturdy Reporting and Debugging: Endowing your test arsenal with sturdy reporting functionality makes it easy to thoroughly analyze test results throughout parallel runs. Debugging failure in the presence of parallel execution requires careful logging and debugging policies.
Conclusion
Running test suites in parallel with Selenium and Java is a game changer, bringing new levels of efficiency and speed to the testing process. With the power of concurrency enabling you to test faster and more accurately, a software testing company will be able to fully embrace testing with speed, fidelity, and confidence without sacrificing quality.
Interested in embracing the philosophy of parallelism, revamping your testing practice, and aspiring for a new level of efficiency and productivity? Hire quality Selenium testing services from PixelQA and get your application tested with accuracy and efficiency. We've got you covered for a smooth user experience.
About Author
With experience of 5+ years as a QA Executive, Gajanan Kolase aspires to rise to a leadership position and become a QA Lead. Started with manual testing, he gradually gained experience in automation testing and advanced quality assurance methodologies. Enjoys learning about new technologies and embracing futuristic trends to stay updated.