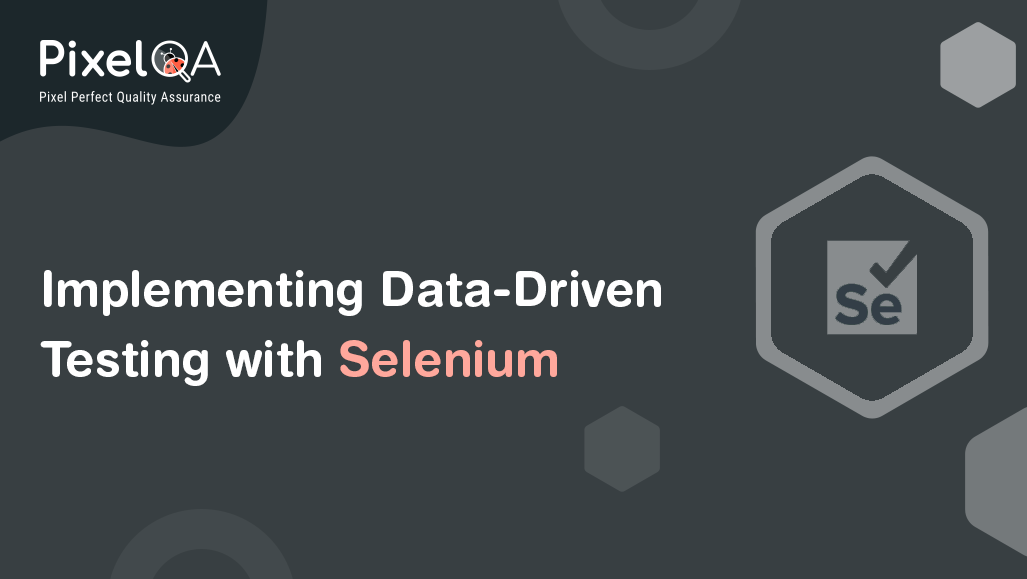
Running test scripts using different data inputs is essential for data-driven testing, which is important for evaluating software performance. This method improves test coverage and efficiency by separating test data from test scripts, enabling the same script to be run with various data inputs multiple times. Selenium automation testing services often leverage this approach to enhance testing accuracy and reusability. This method is particularly useful for confirming application functionality in different scenarios without needing to make multiple test cases.
Data-driven testing uses external data sources such as databases, CSV files, or spreadsheets to make tests reusable, maintainable, and scalable. This approach saves time and effort by increasing test reliability through the exploration of more diverse datasets. Selenium, JUnit, and TestNG are commonly used in modern software development for data-driven testing, a popular practice.
Implementing data-driven testing guarantees comprehensive test coverage and improvements to the software application through various scenarios.
Table of Contents
- What is Data-Driven Testing?
- Advantages of Data-Driven Testing
- Data Sources for Data-Driven Testing
- Implementing Data-Driven Testing with Selenium with steps
- Building Data-Driven Tests with Selenium
- Conclusion
What Does Data-Driven Testing Mean?
Data-driven testing offers a major benefit by simplifying the testing process through the separation of test logic and data. This allows for running many test cases using various data sets, enabling the reuse of the same test script with different inputs multiple times. Consequently, it enhances test coverage and efficiency. Additionally, this approach supports easy maintenance and scalability, as test data can be managed independently of the test scripts, typically being accessed from external sources such as CSV files, Excel sheets, or databases. This flexibility also facilitates the testing of more complex scenarios, ensuring comprehensive validation of the application under diverse conditions.
This method provides numerous advantages:
1. Reusability: Test scripts have the ability to be employed with varied sets of data.
Test script maintenance is simplified as test cases can be easily updated without necessitating alterations to the scripts due to data modifications.
2. Scalability: Additional test scenarios can easily be incorporated by including new sets of data.
3. Enhanced coverage: Ensures testing the application with a diverse set of inputs, revealing additional potential problems.
Utilizing test data logic in data-driven testing enhances the evaluation of web application and software functionality, leading to more intricate and effective validation procedures.
Advantages of Data-Driven Testing
Selenium provides multiple benefits for data-driven testing, improving the efficiency and effectiveness of your testing processes. Here are the top five benefits:
1. Increased Test Coverage:
DDT allows you to execute the same test script with multiple sets of data. This means you can test a wide range of scenarios and edge cases without writing separate test cases for each, thereby increasing your test coverage comprehensively.
2. Reusability and Maintainability:
By separating test data from test scripts, you create reusable components. This reduces redundancy in your test suite and makes maintenance easier. Changes in test data can be made independently of the test scripts, promoting better organization and scalability.
3. Faster Feedback Loop:
With DDT, you can run tests with various datasets in parallel or sequentially, depending on your needs. This parallel execution capability speeds up the testing process, providing faster feedback on the application's behavior under different conditions.
4. Enhanced Flexibility:
Test data can be easily modified or expanded without altering the test scripts themselves. This flexibility allows testers to adapt to changes in requirements or to explore additional scenarios without significant overhead.
5. Enhanced Precision and Dependability:
Automation with DDT lessens the chance of human mistakes in testing by streamlining the input of test data into the testing scripts. This enhances the precision and dependability of test outcomes by reducing the chance of errors from manual data input and maintaining uniform test performance.
Data Sources for Data-Driven Testing
1. CSV (Comma-Separated Values):
Store your test data in CSV files that can be conveniently read with libraries such as OpenCSV or Apache Commons CSV. These files may include basic values separated by commas, which makes them easy to handle.
2. Excel Sheets:
Use Apache POI or JExcelAPI to read data from Excel sheets. Define your test data in Excel files and use Java to read this data and pass it to your Selenium tests.
3. Databases:
Retrieve test data directly from databases using JDBC (Java Database Connectivity). This method is appropriate for situations that require dynamically generating or retrieving test data from a centralized location.
4. Utilize JSON or XML:
Files for storing test data in a well-organized manner. Tools such as Jackson or JAXB are utilized for extracting necessary data by parsing files, like JSON or XML, in libraries.
5. Data Providers:
By utilizing a testing framework such as TestNG or JUnit, you have the ability to develop data provider methods that furnish test data for your test methods. This enables you to maintain a distinction between your test data and test logic.
Implementing Data-Driven Testing with Selenium
Step 1: Set Up Your Project
Ensure you have Selenium WebDriver and TestNG (TestNG is optional but highly recommended for testing in Java) dependencies added to your Java project. You can use Maven or Gradle for dependency management.
Step 2: Prepare Your Test Data
Create your test data in a suitable format. Common formats include CSV, Excel, JSON, or XML. This data will contain the input values for your tests.
Step 3: Read Test Data
Write code to read test data from your chosen format. For instance, when working with CSV files, you have the option to utilize tools such as OpenCSV or Apache Commons CSV for data reading. If you're using Excel, Apache POI is a popular choice.
Step 4: Write Your Test Cases
Write your test cases using TestNG (or JUnit). Use parameterization to pass test data to your test methods. Annotate your test methods with @Test and use parameters to receive test data.
Step 5: Execute Your Tests
Run your tests using a test runner. If you're using TestNG, you can run your tests through TestNG XML files or directly from your IDE. Gradle and Maven also have plugins for running TestNG tests.
Building Data-Driven Tests with Selenium
In this part, we will explore creating tests driven by data with Selenium, emphasizing the effective implementation of this method. Let’s explore the core components of a data-driven test, particularly through the use of the ReadExcel and TestData classes in a Java environment with Eclipse.
1. Setting Up the Environment
To start, ensure that you have your development environment ready. Launch Eclipse and verify that you have the required dependencies, including Selenium WebDriver and Apache POI for Excel file management, integrated into your project. If you are employing Maven or Gradle, make sure to add these dependencies in your pom.xml or build.gradle file, as needed.
2. Creating the ReadExcel Class
The ReadExcel class is responsible for reading data from Excel files. This class will utilize Apache POI to open and parse Excel files, extracting the necessary test data. Below is a basic example of how you can implement the ReadExcel class:
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.FileInputStream;
import java.io.IOException;
public class ReadExcel {
private String filePath;
public ReadExcel(String filePath) {
this.filePath = filePath;
}
public Object[][] getTestData() throws IOException {
FileInputStream fileInputStream = new FileInputStream(filePath);
Workbook workbook = new XSSFWorkbook(fileInputStream);
Sheet sheet = workbook.getSheetAt(0);
int rowCount = sheet.getPhysicalNumberOfRows();
int colCount = sheet.getRow(0).getPhysicalNumberOfCells();
Object[][] data = new Object[rowCount - 1][colCount];
for (int i = 1; i < rowCount; i++) {
Row row = sheet.getRow(i);
for (int j = 0; j < colCount; j++) {
data[i - 1][j] = row.getCell(j).toString();
}
}
workbook.close();
fileInputStream.close();
return data;
}
}
3. Implementing the TestData Class
The TestData class is where you define the test cases and integrate the test data read by the ReadExcel class. This class will use TestNG annotations to run the tests with the data provided. Here’s a basic example:
import org.testng.annotations.DataProvider;
import org.testng.annotations.Test;
public class TestData {
@DataProvider(name = "data-provider")
public Object[][] dataProvider() throws IOException {
ReadExcel readExcel = new ReadExcel("path/to/your/excel/file.xlsx");
return readExcel.getTestData();
}
@Test(dataProvider = "data-provider")
public void testMethod(String input1, String input2, String expectedResult) {
// Implement your test logic here using the provided data
System.out.println("Input 1: " + input1);
System.out.println("Input 2: " + input2);
System.out.println("Expected Result: " + expectedResult);
// Your test assertions and actions
}
}
4. Running the Tests
Once you have your ReadExcel and TestData classes set up, you can run your tests using TestNG. You can execute the tests directly from Eclipse or through the command line if you are using Maven or Gradle. Ensure your test data file is correctly placed and accessible to avoid file-related issues.
Conclusion
Utilizing data-driven testing with Selenium provides a strong approach to testing web applications by separating test data from scripts. This method boosts test coverage, encourages reusability, and improves flexibility and maintainability. By utilizing various data sources and implementing parameterization techniques, testers can efficiently execute tests with different datasets, ensuring thorough validation of application functionality. A software testing company can streamline this process by implementing best practices and tools tailored for scalability. Despite challenges such as data source management and test maintenance, solutions like modularization and custom reporting mitigate these issues. Incorporating data-driven tests into CI/CD pipelines enables prompt evaluation of application modifications, ultimately enhancing the dependability and effectiveness of the testing procedure.
About Author
Vedant Parmar is a veteran QA executive who believes in continuous learning, training, and acquiring new skills. He wants to pursue a career in Mobile Test Automation and Penetration Testing and strive to be a QA manager in the professional journey.