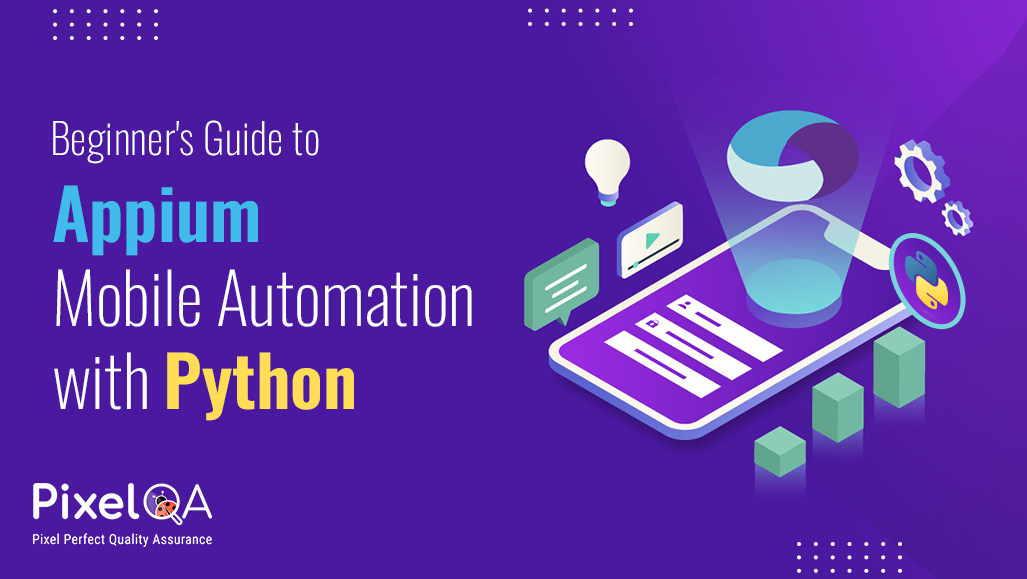
Data-driven testing is necessary when evaluating software performance by applying different data inputs to run test scripts. It improves testing coverage and efficiency since test data can be separate from the scripts, so that the same script can run again and again with different data inputs. This is especially valuable for confirming functionality of the application in many different scenarios without having to create multiple test cases, especially in Mobile App Testing Services to check broad device and platform compatibility.
Data-driven testing generally refers to getting external sources, such as databases, CSV files, and spreadsheets, to make tests reusable, maintainable, and scalable. The use of external data, DBs, CSV files, or spreadsheets makes the tests more reliable in that they tend to use more diverse sets of data and save huge amounts of time and resources. Most of the software data-driven test scripts being used today are based on Selenium, JUnit, and testing. Implementing data-driven testing guarantees comprehensive test coverage and improvements to the software application through various scenarios.
Table of Contents
- What is Data-Driven Testing?
- Advantages of Data-Driven Testing
- Data Sources for Data-Driven Testing
- Implementing Data-Driven Testing with Selenium with steps
- Building Data-Driven Tests with Selenium
- Conclusion
What Does Data-Driven Testing Mean?
Data-driven testing allows for a notable benefit by streamlining the test process. Through separating test logic from data. Through this, there is the possibility of running a large number of test cases on various sets of data, while the same script is used time and again, using different inputs. This improves test coverage and efficiency. Further, this method facilitates simple maintenance and scalability since test data can be handled separately from the test scripts, usually accessed from outside resources like CSV files, Excel files, or databases. This freedom also allows more complex scenarios to be tested, providing thorough validation of the application under various circumstances.
This method provides numerous advantages:
- Reusability: Test scripts have the ability to be employed with varied sets of data. Test script maintenance is simplified as test cases can be easily updated without necessitating alterations to the scripts due to data modifications.
- Scalability: Additional test scenarios can easily be incorporated by including new sets of data.
- Enhanced coverage: Ensures testing the application with a diverse set of inputs, revealing additional potential problems.
More complex and efficient validation processes result from the use of test data logic in data-driven testing, which improves the assessment of software and web application functioning.
Advantages of Data-Driven Testing
Selenium enhances the efficacy and efficiency of your testing procedures. By offering a number of advantages for data-driven testing. Here are the top five benefits:
1. Increased Test Coverage:
DDT enables you to run the same test script with different sets of data. This implies that you can test a large number of scenarios and edge cases. Without creating individual test cases for each, thus expand your test coverage in depth.
2. Reusability and Maintainability:
By decoupling test data from test scripts, you have reusable pieces. This decreases redundancy in your test suite and simplifies maintenance. Test data changes can be done independently of the test scripts, allowing for better organization and scalability.
3. Faster Feedback Loop:
With DDT, you can test with different sets of data in parallel or sequential modes. Depending on your requirements. This parallel execution feature accelerates the testing process. and you get faster feedback on the behavior of the application with different inputs.
4. Enhanced Flexibility:
Test data is a simple matter to change or expand without affecting the test scripts themselves. Because of this, testers can easily accommodate changes in requirements or try out different scenarios without too much overhead.
5. Enhanced Precision and Dependability:
Automation with DDT lessens the chance of human mistakes in testing. by streamlining the input of test data into the testing scripts. This enhances the precision and dependability of test outcomes. By reducing the chance of errors from manual data input and maintaining uniform test performance.
Data Sources for Data-Driven Testing
1. CSV (Comma-Separated Values):
Store your test data in CSV files which are easily readable using libraries like OpenCSV or Apache Commons CSV. The files can have simple values comma-separated, and hence are easy to work with.
2. Excel Sheets:
Use Apache POI or JExcelAPI to read data from Excel sheets. Your test data should be defined in Excel files, which Java may then read and send to your Selenium tests.
3. Databases:
JDBC (Java Database Connectivity) allows you to retrieve test data straight from databases. When test data needs to be generated dynamically or retrieved from a central place, this approach is suitable.
4. Utilize JSON or XML:
Files for storing test data in a well-organized manner. Tools such as Jackson or JAXB are utilized for extracting necessary data by parsing files, like JSON or XML, in libraries.
5. Data Providers:
By using a test framework like TestNG or JUnit, you can create data provider methods that provide test data. This allows you to keep your test data and test logic separate.
Implementing Data-Driven Testing with Selenium
Step 1: Set Up Your Project
Be sure that your Java project has dependencies on Selenium WebDriver and TestNG (TestNG is an optional dependency, but it is certainly recommended for any kind of testing in Java). You can add those dependencies using either Maven or Gradle.
Step 2: Prepare Your Test Data
Create your test data in a suitable format. Common formats include CSV, Excel, JSON, or XML. This data will contain the input values for your tests.
Step 3: Read Test Data
Write code to read test data from your chosen format. For example, when you're dealing with CSV files, you can have the option to use libraries. like OpenCSV or Apache Commons CSV for reading data. If you're working in Excel, Apache POI is commonly used.
Step 4: Write Your Test Cases
Write your test cases using TestNG (or JUnit). Use parameterization to pass test data to your test methods. Annotate your test methods with @Test and use parameters to receive test data.
Step 5: Execute Your Tests
Execute your tests in a test runner. If you're working with TestNG, you can execute your tests by TestNG XML files or run them directly from your IDE. Gradle and Maven also have TestNG test-running plugins.
Building Data-Driven Tests with Selenium
In this part, we will explore creating tests driven by data with Selenium, emphasizing the effective implementation of this method. Let’s explore the core components of a data-driven test, particularly through the use of the ReadExcel and TestData classes in a Java environment with Eclipse.
1. Setting Up the Environment
First, have your development environment set up. Open Eclipse and ensure that you have the dependencies necessary. Such as Selenium WebDriver and Apache POI for managing Excel files, included in your project. If you are using Maven or Gradle, ensure that you include these dependencies in your pom.xml or build.gradle file, respectively.
2. Creating the ReadExcel Class
The ReadExcel class will be used to read data from Excel sheets. The ReadExcel class will use Apache POI to read and parse Excel sheets and pull out the required test data. Here is a simple example of how you can make the ReadExcel class:
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.FileInputStream;
import java.io.IOException;
public class ReadExcel {
private String filePath;
public ReadExcel(String filePath) {
this.filePath = filePath;
}
public Object[][] getTestData() throws IOException {
FileInputStream fileInputStream = new FileInputStream(filePath);
Workbook workbook = new XSSFWorkbook(fileInputStream);
Sheet sheet = workbook.getSheetAt(0);
int rowCount = sheet.getPhysicalNumberOfRows();
int colCount = sheet.getRow(0).getPhysicalNumberOfCells();
Object[][] data = new Object[rowCount - 1][colCount];
for (int i = 1; i < rowCount; i++) {
Row row = sheet.getRow(i);
for (int j = 0; j < colCount; j++) {
data[i - 1][j] = row.getCell(j).toString();
}
}
workbook.close();
fileInputStream.close();
return data;
}
}
3. Implementing the TestData Class
The TestData class is where you define the test cases and integrate the test data read by the ReadExcel class. This class will use TestNG annotations to run the tests with the data provided. Here’s a basic example:
import org.testng.annotations.DataProvider;
import org.testng.annotations.Test;
public class TestData {
@DataProvider(name = "data-provider")
public Object[][] dataProvider() throws IOException {
ReadExcel readExcel = new ReadExcel("path/to/your/excel/file.xlsx");
return readExcel.getTestData();
}
@Test(dataProvider = "data-provider")
public void testMethod(String input1, String input2, String expectedResult) {
// Implement your test logic here using the provided data
System.out.println("Input 1: " + input1);
System.out.println("Input 2: " + input2);
System.out.println("Expected Result: " + expectedResult);
// Your test assertions and actions
}
}
4. Running the Tests
After you have your ReadExcel and TestData classes ready, you can execute your tests through TestNG. You can run the tests within Eclipse or command line if you are using Maven or Gradle. Your test data file should be properly located and accessible so as not to encounter any issues of a file nature.
Conclusion
A data-driven testing approach using Selenium provides a strong format for testing web applications, isolating test data from scripts. The technique permits huge test coverage, enhances reusability, and thereby increases flexibility and maintainability. With the use of various data sources and parameterization methods for tests, testers may run multiple datasets efficiently for proper application function validity. The challenges of data source management and test maintenance notwithstanding, a Software Testing Company can implement solutions such as modularization and customized reporting to help counteract these. Integrating data-driven tests into CI/CD pipelines allows for the timely assessment of application changes, thereby improving the reliability and efficiency of the testing process.
About Author
Vedant Parmar is a veteran QA executive who believes in continuous learning, training, and acquiring new skills. He wants to pursue a career in Mobile Test Automation and Penetration Testing and strives to be a QA manager in his professional journey.