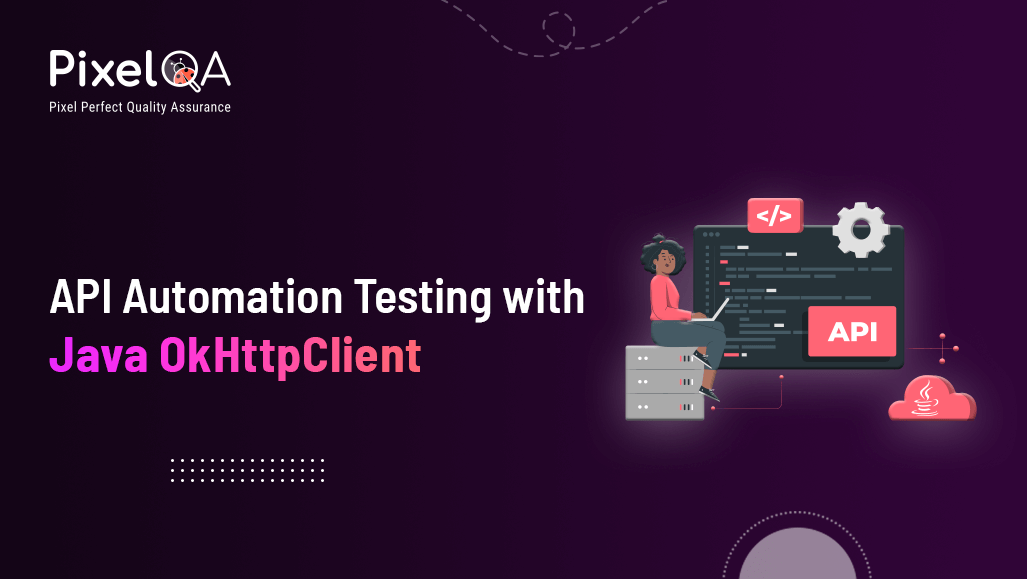
Introduction
The seamless functioning of APIs is essential in the quick-paced industry of software development. To achieve this, API testing services and automation testing are essential since they enable teams to quickly examine endpoints, evaluate response data, and confirm performance. Java OkHttpClient stands out as a strong option among the many tools available for API testing, providing flexibility, dependability, and user-friendliness. We examine the benefits, implementation techniques, and best practices of Java OkHttpClient as we delve into the nuances of API automation testing with this extensive article.
Table of Contents
- Introduction
- Understanding API Automation Testing
- Introducing Java OkHttpClient
- Getting Started with Java OkHttpClient and TestNG
- Best Practices for API Automation Testing with TestNG
- Benefits of Selenium and OkHttp Integration
- Conclusion
Understanding API Automation Testing
The basis of current software applications is APIs, which enable data interchange and communication across various platforms. Testing APIs entails confirming their security, performance, dependability, and functionality. In this sense, automation testing involves the process of automatically running test cases to assure correct and consistent results—without the need for human interaction.
API automation testing includes several elements, such as:
- Functional Testing: Validating the behavior of API endpoints against specified requirements and expected outcomes.
- Integration Testing: The process of using API calls to confirm how various application components interact with one another.
- Regression Testing: Validating that modifications or improvements to the API do not negatively impact currently available functionality.
- Load Testing: The process of verifying an API's speed and capacity under varied load conditions.
- Security Testing: The process of identifying weaknesses and verifying that everything meets security policies and procedures.
Introducing Java OkHttpClient
Java OkHttpClient is a robust HTTP client for Java-based programs that offers a user-friendly interface for handling responses and requesting information via HTTP. Constructed upon the foundation of OkHttp, a well-known Java HTTP client library, it offers an extensive array of functionalities for engaging with RESTful APIs, such as:
- Efficient Connection Pooling: OkHttpClient oversees connection reuse and pooling, maximizing efficiency and minimizing latency.
- Support for HTTP/2 and SPDY: It seamlessly supports modern HTTP protocols, enabling faster and more efficient communication.
- Interceptor Architecture: Interceptors allow developers to modify requests and responses, add authentication, logging, or custom headers, and handle errors effectively.
- Asynchronous Requests: OkHttpClient allows for parallel request handling by using Completable Future or callbacks for offering non-blocking input/output activities.
- WebSocket Support: Its inbuilt WebSocket communication capabilities allow real-time bidirectional data transfer.
- MockWebServer for Testing: The MockWebServer module provides a portable, scriptable HTTP server for testing HTTP clients, making it ideal for API testing.
Getting Started with Java OkHttpClient and TestNG
Step 1: Setting Up the Project
Start by creating a new Maven project and adding dependencies for TestNG and OkHttpClient to your project's build configuration file.
Example:
<dependency>
<groupId>org.testng</groupId>
<artifactId>testng</artifactId>
<version>7.4.0</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>com.squareup.okhttp3</groupId>
<artifactId>okhttp</artifactId>
<version>4.9.0</version>
</dependency>
Step 2: Writing Test Cases with TestNG
Define test cases using TestNG annotations such as `@Test`, `@BeforeClass`, `@AfterClass`, etc. Each test method should represent a specific API endpoint or functionality that needs to be tested.
Example:
import okhttp3.*;
import org.testng.annotations.Test;
import static org.testng.Assert.*;
public class APITest {
private final OkHttpClient client = new OkHttpClient();
@Test
public void testGetRequest() throws Exception {
Request request = new Request.Builder()
.url("https://api.example.com/users/1")
.build();
try (Response response = client.newCall(request).execute()) {
assertEquals(200, response.code());
assertEquals("application/json", response.header("Content-Type"));
// Add more assertions as needed
}
}
// Add more test cases for different endpoints and scenarios
}
Step 3: Handling Authentication
If your API requires authentication, utilize OkHttp's authentication mechanisms to include credentials in your HTTP requests securely.
Example:
import okhttp3.Credentials;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.Response;
public class APITestWithAuth {
public void auth throws Exception {
String username = "your_username";
String password = "your_password";
String credentials = Credentials.basic(username, password);
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url("https://api.example.com/data")
.header("Authorization", credentials)
.build();
try (Response response = client.newCall(request).execute()) {
System.out.println(response.body().string());
}
}
}
Step 4: Running Tests with TestNG
Execute the test cases using TestNG's test runner, either from the command line or your IDE. TestNG will automatically discover and execute the test methods annotated with `@Test`.
Best Practices for API Automation Testing with TestNG
- Organize Test Suites: Group related test cases into test suites using TestNG XML configuration files for better organization and management.
- Use Data Providers: Utilize TestNG's `@DataProvider` annotation to supply test data dynamically and parameterize test methods.
- Handle Dependencies: Use TestNG's `dependsOnMethods` and `dependsOnGroups` attributes to handle test method dependencies and execution order.
- Leverage Asserts: Use TestNG's built-in assertions for validating expected outcomes and ensuring test case reliability.
- Use TestNG Listeners: Configure TestNG listeners to manage test lifecycle events, generate complete test results, and adjust test execution behavior.
- Parallel Execution: For faster test execution and to boost overall effectiveness, make use of TestNG's parallel execution features.
Benefits of Selenium and OkHttp Integration
The integration of Selenium WebDriver and OkHttp offers several benefits for API automation testing:
- Unified Testing Framework: By using existing Selenium test suites, developers can easily integrate API testing, simplifying the procedure and reducing overhead.
- Robustness and Flexibility: Testers may use a robust and adaptable automation framework that can handle a broad range of testing situations and needs by combining the qualities of Selenium and OkHttp.
- Efficiency and Scalability: By automating repetitive operations and permitting parallel test execution, automation testing using Selenium and OkHttp improves testing efficiency and speeds up the testing process while also increasing overall productivity.
Conclusion
Developers and testers may design strong and thorough API automation test suites by combining the flexibility and power of TestNG with the resilience of Java OkHttpClient. This makes it possible to thoroughly validate API endpoints, guarantee that specifications are followed, and find possible problems early in the development cycle. A Software testing company can leverage these tools to help teams confidently optimize product quality, offer outstanding user experiences, and expedite API testing procedures by utilizing TestNG's extensive feature set in combination with Java OkHttpClient's efficient HTTP client capabilities.
About Author
With experience of 5+ years as a QA Executive, Gajanan Kolase aspires to rise to a leadership position and become a QA Lead. Started with manual testing, he gradually gained experience in automation testing and advanced quality assurance methodologies. Enjoys learning about new technologies and embracing futuristic trends to stay updated.